Compute Median of Image at Pixel#
Synopsis#
Computes the median of an image at a pixil(in a regular neighborhood).
Results#
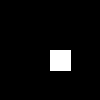
input.png#
Output:
Median at [10, 10] is 0
Code#
C++#
#include "itkImage.h"
#include "itkImageFileWriter.h"
#include "itkImageRegionIterator.h"
#include "itkMedianImageFunction.h"
using UnsignedCharImageType = itk::Image<unsigned char, 2>;
void
CreateImage(UnsignedCharImageType::Pointer image);
int
main()
{
auto image = UnsignedCharImageType::New();
CreateImage(image);
using MedianImageFunctionType = itk::MedianImageFunction<UnsignedCharImageType>;
auto medianImageFunction = MedianImageFunctionType::New();
medianImageFunction->SetInputImage(image);
itk::Index<2> index;
index.Fill(10);
std::cout << "Median at " << index << " is " << static_cast<int>(medianImageFunction->EvaluateAtIndex(index))
<< std::endl;
return EXIT_SUCCESS;
}
void
CreateImage(UnsignedCharImageType::Pointer image)
{
itk::Index<2> start;
start.Fill(0);
itk::Size<2> size;
size.Fill(100);
itk::ImageRegion<2> region(start, size);
image->SetRegions(region);
image->Allocate();
image->FillBuffer(0);
itk::ImageRegionIterator<UnsignedCharImageType> imageIterator(image, region);
while (!imageIterator.IsAtEnd())
{
if (imageIterator.GetIndex()[0] >= 50 && imageIterator.GetIndex()[1] >= 50 && imageIterator.GetIndex()[0] <= 70 &&
imageIterator.GetIndex()[1] <= 70)
{
imageIterator.Set(255);
}
++imageIterator;
}
itk::WriteImage(image, "input.png");
}
Classes demonstrated#
-
template<typename TInputImage, typename TCoordRep = float>
class MedianImageFunction : public itk::ImageFunction<TInputImage, TInputImage::PixelType, TCoordRep> Calculate the median value in the neighborhood of a pixel.
Calculate the median pixel value over the standard 8, 26, etc. connected neighborhood. This calculation uses a ZeroFluxNeumannBoundaryCondition.
If called with a ContinuousIndex or Point, the calculation is performed at the nearest neighbor.
This class is templated over the input image type and the coordinate representation type (e.g. float or double ).
- ITK Sphinx Examples: